个人博客
http://www.milovetingting.cn
Android自定义View-圆形图片控件
前言
在日常开发中,圆形的图片效果还是很常见的。可以通过给Paint
设置Xfermode
来实现,这里简单记录如下。
实现
实现圆形效果的核心是PorterDuffXfermode
,对于PorterDuffXfermode,这里不展开,可以查询相关资料。
核心代码
1 2 3 4 5 6 7 8
| canvas.drawCircle(mSize / 2, mSize / 2, mSize / 2, mPaint);
mPaint.setXfermode(new PorterDuffXfermode(PorterDuff.Mode.SRC_IN));
canvas.drawBitmap(mSrcBitmap, 0, 0, mPaint);
mPaint.setXfermode(null);
|
自定义属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name="CircleView"> <attr name="src" format="reference" /> <attr name="type" format="enum"> <enum name="round" value="1" /> <enum name="rect" value="2" /> </attr> </declare-styleable> </resources>
|
自定义控件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112
| public class CircleView extends View {
private static final int DEFAULT_SIZE = 200;
private static final int DEFAULT_RADIUS = 20;
private static final int TYPE_ROUND = 1;
private static final int TYPE_RECT = 2;
private int mSize;
private int mResourceId;
private int mType;
private Paint mPaint;
private Bitmap mSrcBitmap;
public CircleView(Context context) { this(context, null); }
public CircleView(Context context, AttributeSet attrs) { this(context, attrs, 0); }
public CircleView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); TypedArray ta = context.obtainStyledAttributes(attrs, R.styleable.CircleView); mResourceId = ta.getResourceId(R.styleable.CircleView_src, R.mipmap.ic_launcher); mType = ta.getInt(R.styleable.CircleView_type, TYPE_ROUND); ta.recycle(); init(); }
@Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { int width = getMeasureSize(widthMeasureSpec); int height = getMeasureSize(heightMeasureSpec); mSize = Math.min(width, height); setMeasuredDimension(mSize, mSize); }
@RequiresApi(api = Build.VERSION_CODES.LOLLIPOP) @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); if (mSrcBitmap == null) { mSrcBitmap = getScaleBitmap(); } if (mType == TYPE_ROUND) { canvas.drawCircle(mSize / 2, mSize / 2, mSize / 2, mPaint); } else if (mType == TYPE_RECT) { canvas.drawRoundRect(0, 0, mSize, mSize, DEFAULT_RADIUS, DEFAULT_RADIUS, mPaint); } mPaint.setXfermode(new PorterDuffXfermode(PorterDuff.Mode.SRC_IN)); canvas.drawBitmap(mSrcBitmap, 0, 0, mPaint); mPaint.setXfermode(null); }
private void init() { setLayerType(LAYER_TYPE_HARDWARE, null); mPaint = new Paint(); mPaint.setAntiAlias(true); mPaint.setStyle(Paint.Style.FILL); }
private int getMeasureSize(int measureSpec) { int mode = MeasureSpec.getMode(measureSpec); int size = MeasureSpec.getSize(measureSpec); return mode == MeasureSpec.EXACTLY ? size : DEFAULT_SIZE; }
private Bitmap getScaleBitmap() { BitmapFactory.Options options = new BitmapFactory.Options(); options.inJustDecodeBounds = true; BitmapFactory.decodeResource(getResources(), mResourceId, options); options.inSampleSize = calcSampleSize(options, mSize, mSize); options.inJustDecodeBounds = false; return BitmapFactory.decodeResource(getResources(), mResourceId, options); }
private int calcSampleSize(BitmapFactory.Options option, int width, int height) { int originWidth = option.outWidth; int originHeight = option.outHeight; int sampleSize = 1; while ((originWidth = originWidth >> 1) > width && (originHeight = originHeight >> 1) > height) { sampleSize = sampleSize << 1; } return sampleSize; } }
|
注意:如果没有圆形的效果,那么可能需要禁用硬件加速:setLayerType(LAYER_TYPE_HARDWARE, null)
布局
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center_horizontal" android:orientation="vertical" tools:context=".MainActivity">
<com.wangyz.custom.CircleView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="10dp" app:src="@drawable/image" />
<com.wangyz.custom.CircleView android:layout_width="100dp" android:layout_height="100dp" android:layout_margin="10dp" app:src="@drawable/image" />
<com.wangyz.custom.CircleView android:layout_width="100dp" android:layout_height="100dp" android:layout_margin="10dp" app:src="@drawable/image" app:type="rect" />
</LinearLayout>
|
效果
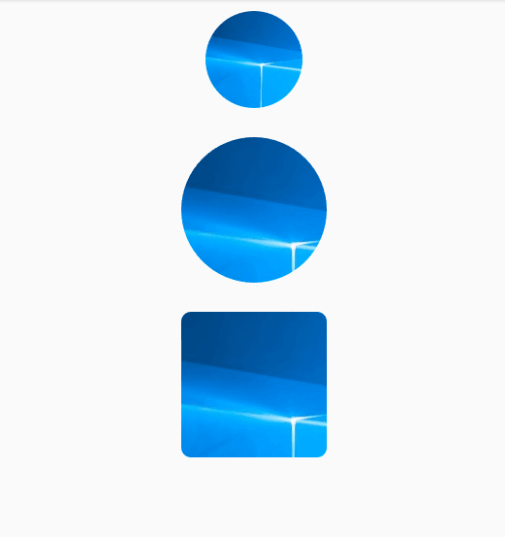